Getting started with Git and Github
Git Command Summary
Git task | Notes | Git commands |
Create a working copy of a local repository: | git clone /path/to/repository | |
For a remote server, use: | git clone username@host:/path/to/repository | |
Commit changes to head (but not yet to the remote repository): | git commit -m "Commit message" | |
Commit any files you've added with git add, and also commit any files you've changed since then: | git commit -a | |
Fetch | Downloads changes from remote repository into local repo. | git push origin master git push <remote-name> <branch-name> |
Push | Write changes up to the master branch of your remote repository: | git push origin master git push <remote-name> <branch-name> |
Status | List the files you've changed and those you still need to add or commit: | git status |
If you haven't connected your local repository to a remote server, add the server to be able to push to it: | git remote add origin <server> | |
List all currently configured remote repositories: | git remote -v | |
Show list of branches for a remote repository | git remote show <repository> git remote show origin |
|
Create a new branch and switch to it: | git checkout -b <branchname> | |
Switch from one branch to another: | git checkout <branchname> | |
List all the branches in your repo, and also tell you what branch you're currently in: | git branch | |
Delete the feature branch: | git branch -d <branchname> /*safe*/ git branch -d <branchname> /*force*/ |
|
Push the branch to your remote repository, so others can use it: | git push origin <branchname> | |
Push all branches to your remote repository: | git push --all origin | |
Delete a branch on your remote repository: | git push origin :<branchname> | |
Fetch and merge changes on the remote server to your working directory: | git pull | |
To merge a different branch into your active branch: | git merge <branchname> | |
View all the merge conflicts:View the conflicts against the base file: Preview changes, before merging: |
git diffgit diff --base <filename> git diff <sourcebranch> <targetbranch> |
|
After you have manually resolved any conflicts, you mark the changed file: | git add <filename> | |
If you mess up, you can replace the changes in your working tree with the last content in head:Changes already added to the index, as well as new files, will be kept. | git checkout -- <filename> | |
Instead, to drop all your local changes and commits, fetch the latest history from the server and point your local master branch at it, do this: | git fetch origin git reset --hard origin/master |
|
Search | Search the working directory for foo(): | git grep "foo()" |
Pull Request Tutorial
Pull Requests are commonly used by teams and organizations collaborating using the Shared Repository Model, where everyone shares a single repository and topic branches are used to develop features and isolate changes. Many open source projects on Github use pull requests to manage changes from contributors as they are useful in providing a way to notify project maintainers about changes one has made and in initiating code review and general discussion about a set of changes before being merged into the main branch. See article here
Git Pull Request Example
cd /repos git checkout master git pull #fast forward git checkout -b <username>/<branchname> vim <filename> git status git add . git status git commit -am "<branch change desc>" git status git pull git push --set-upstream origin <username>/<branchname> https://github.com/<project>/<repos>/pulls (New pull request) git checkout master git pull
Git Basics
Command | Description |
---|---|
$git init <name> | Initialize Local Git <name> Repository for current directory, creates .git directory |
$git add <file> | Add files to index i.e. *.php or git add . will add all files |
$git commit -m 'message' | Commit changes in index to local repository (-m) with commit message |
$git status | Show what is in the staging area |
$git diff --stagged | Show file differences between staging and the last file version |
$touch .gitignore | Ignore this list of files or patterns not to add to repository |
$git show | Alias for git log -p -n 1 |
$git log | Show recent activity |
$git reflog | show ref log history |
$git remote | Show remote repositories |
$git remote add origin URL | Add a remote repository, like from Github |
$git push -u origin master | Push to remote repository, will prompt for login to github |
$git pull | Clone repository into a new directory |
Git Branching
Command | Description |
---|---|
$git branch | Show branches |
$git branch <mybranch> | Create a new branch, does not change to the new branch |
$git checkout <mybranch> | Switch to the <mybranch> branch |
$git git merge <mybranch> | Merge the changes in <mybranch> back into Master branch |
Git Plumbing
Command | Description |
---|---|
$git cat-file <SHA1> | Show content (-t) type (-s) size (-p) pretty, of objects in repository |
$git hash-object | Calculate SHA1 hash of object or file, optionally create blob from file |
$git count-objects -H | Count unpacked number of objects (-H) human readable |
Git Alias
You can use git aliases, e.g.
git config --global alias.add-commit '!git add -A && git commit'
and use it with
git add-commit -m 'My commit message'
Introduction and Installation
Difference between Git and any other VCS
Git Overview Slides
Git Cheat Sheet1 Sheet2
Install Git
GitHub provides desktop clients that include a graphical user interface for the most common repository actions and an automatically updating command line edition of Git for advanced scenarios.
GitHub Desktop
https://desktop.github.com/
Git distributions for Linux and POSIX systems are available on the official Git SCM website.
Git for all platforms
http://git-scm.com
Git Structure
Git Workflow
Git Contribute to Project
Full Git Reference
Configure tooling
Configure user information for all local repositories
$ git config --global user.name "[name]"
Sets the name you want attached to your commit transactions
$ git config --global user.email "[email address]"
Sets the email you want attached to your commit transactions
Create repositories
Start a new repository or obtain one from an existing URL
$ git init [project-name]
Creates a new local repository with the specified name
$ git clone [url]
Downloads a project and its entire version history
Make changes
Review edits and craft a commit transaction
$ git status
Lists all new or modified files to be committed
$ git diff
Shows file differences not yet staged
$ git add [file]
Snapshots the file in preparation for versioning
$ git diff --staged
Shows file differences between staging and the last file version
$ git reset [file]
Unstages the file, but preserves its contents
$ git commit -m"[descriptive message]"
Records file snapshots permanently in version history
Group changes
Name a series of commits and combine completed efforts
$ git branch
Lists all local branches in the current repository
$ git branch [branch-name]
Creates a new branch
$ git checkout [branch-name]
Switches to the specified branch and updates working directory
$ git merge [branch-name]
Combines the specified branch’s history into the current branch
$ git branch -d [branch-name]
Deletes the specified branch
Refactor file names
Relocate and remove versioned files
$ git rm [file]
Deletes the file from the working directory and stages the deletion
$ git rm --cached [file]
Removes the file from version control but preserves the file locally
$ git mv [file-original] [file-renamed]
Changes the file name and prepare it for commit
Suppress tracking
Exclude temporary files and paths
*.log build/ temp-*
A text file named .gitignore suppresses accidental versioning of files and paths matching the specified patterns
$ git ls-files --others --ignored --exclude-standard
Lists all ignored files in this project
Save fragments
Shelve and restore incomplete changes
$ git stash
Temporarily stores all modified tracked files
$ git stash pop
Restores the most recently stashed files
$ git stash list
Lists all stashed changesets
$ git stash drop
Discards the most recently stashed changeset
Review history
Browse and inspect the evolution of project files
$ git log
Lists version history for the current branch
$ git log --follow [file]
Lists version history for the file, including renames
$ git diff [first-branch]...[second-branch]
Shows content differences between two branches
$ git show [commit]
Outputs metadata and content changes of the specified commit
Redo commits
Erase mistakes and craft replacement history
$ git reset [commit]
Undoes all commits after [commit], preserving changes locally
$ git reset --hard [commit]
Discards all history and changes back to the specified commit
Synchronize changes
Register a remote (URL) and exchange repository history
$ git fetch [remote]
Downloads all history from the remote repository
$ git merge [remote]/[branch]
Combines the remote branch into the current local branch
$ git push [remote] [branch]
Uploads all local branch commits to GitHub
$ git pull
Downloads bookmark history and incorporates changes
Other Tools
When you install Git, you also get its visual tools, gitk
Images
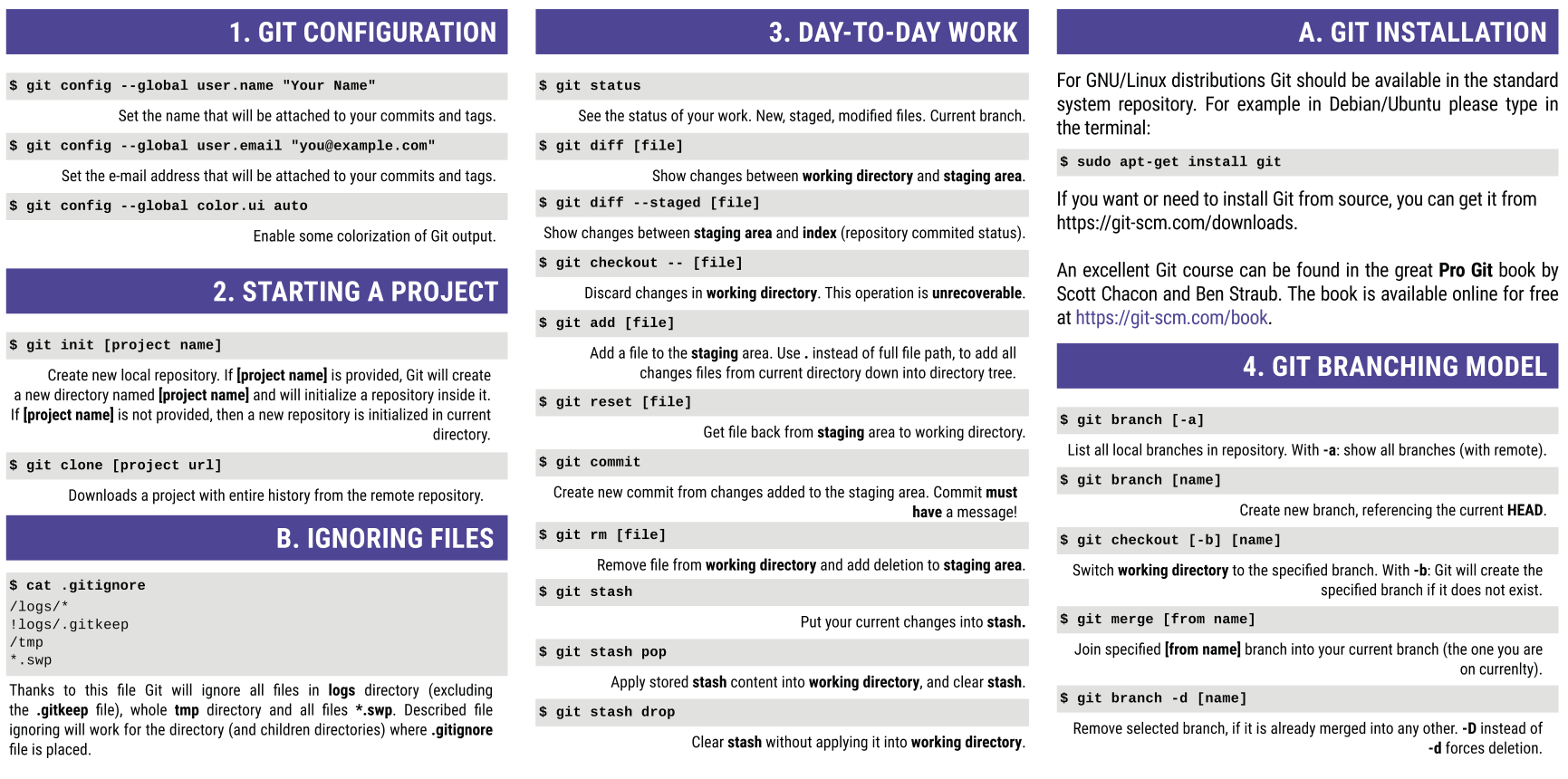
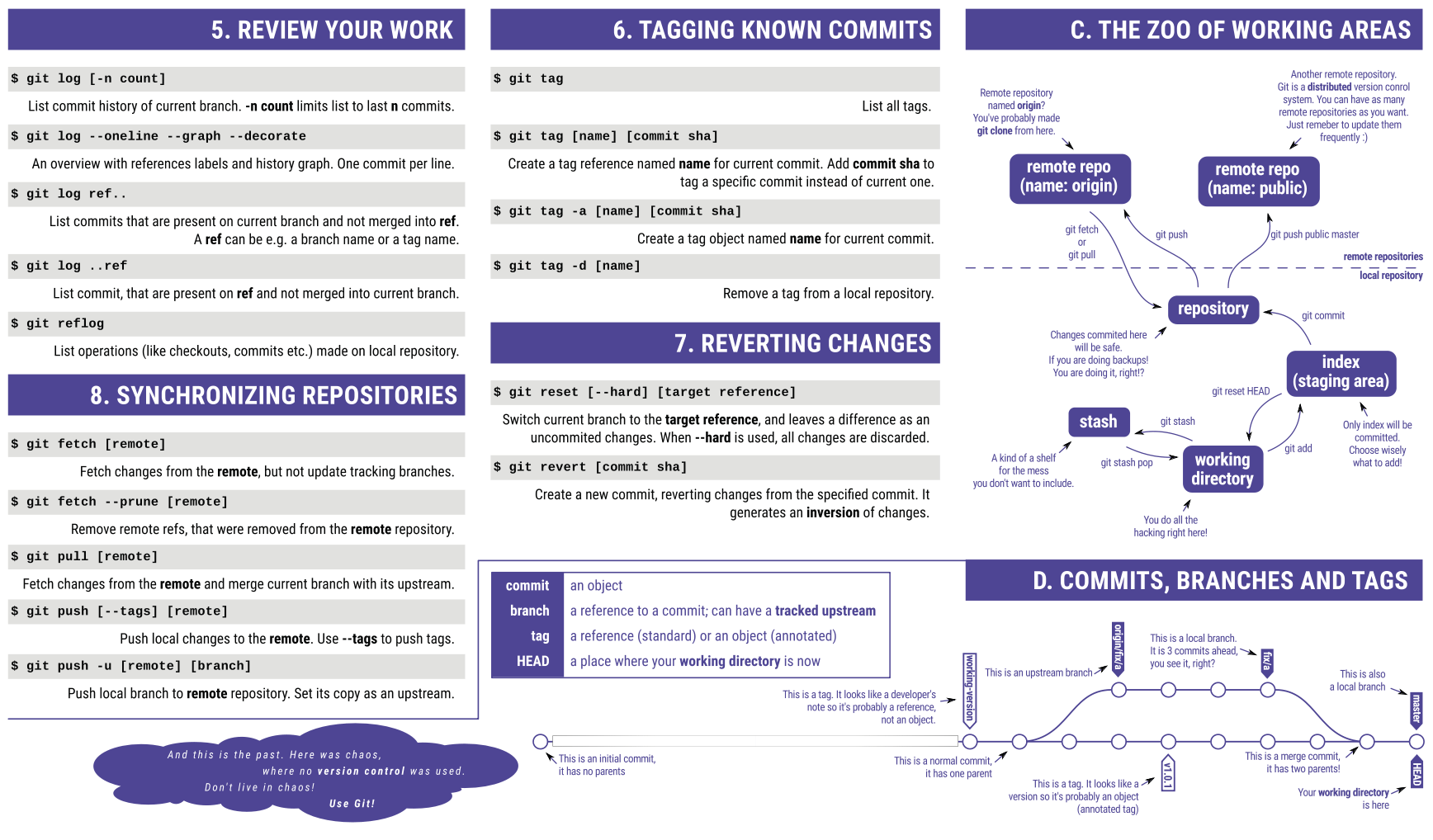